Never, ever, ever, ever, ever give up
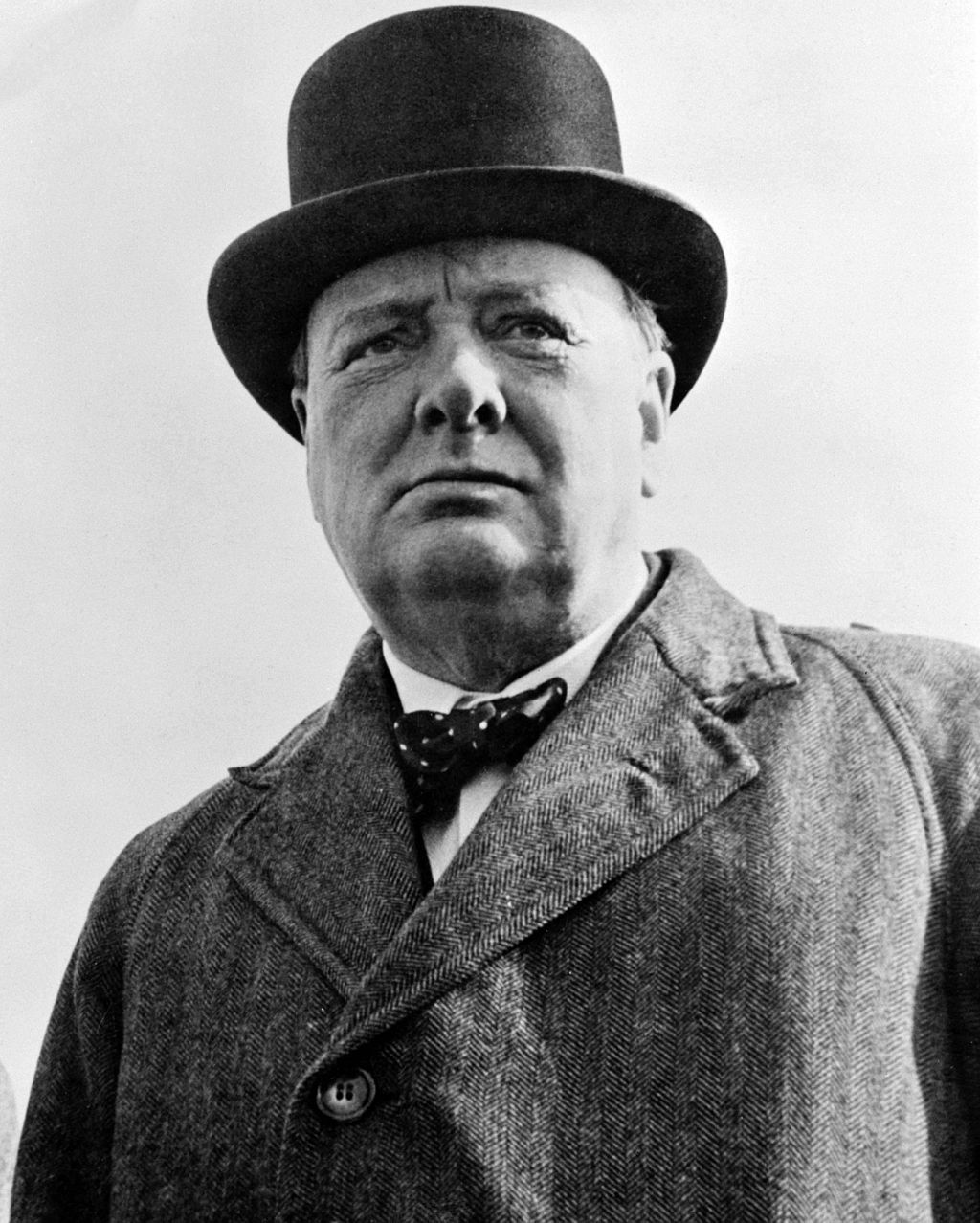
Just don’t do it. You’ve come so far. Don’t believe that just because you’re stuck you can never make it through.
Part of my work at the moment is as a solo indie developer. I’ve built Sol Trader on my own with the help of an artist and lots of encouragement from friends and family. It’s been incredibly tough. The Kickstarter campaign I spent so much time working on didn’t reach its goal, despite tons of interest. But the very same week the game was greenlit by Steam - imagine if I’d thrown in the towel a day earlier?
If you’re a indie developer like me, remind yourself of why you got into this in the first place. Look at what you’ve achieved so far. Look at the old screenshots of your game, and compare them to what you’ve got now. Copy/paste encouraging comments and notes from forums onto your computer somewhere and read through them regularly. Ignore haters. There’s no way they could achieve what you’re going to achieve.
Don’t know how to continue? Try the formula for motivation:
- Break the task down into smaller more manageable chunks
- Raise your confidence you can do it. Read this post twice each morning. Get a mentor to help you with the tough bits.
- Remind yourself why you’re doing this. Every day, if necessary.
- Aggressively reduce distractions: Turn off twitter, facebook, news sites, news in general, everything. Use the pomodoro technique if you need to.
- Commit to do a small part of the task today, or a tiny part before the hour is up.
Creativity is hard. We get the first 90% through, then we hit the second 90%. It’s so easy to give up, but we might miss our greatest achievement.
Sure, if you need to make a positive decision to move on from something that isn’t working, that’s fine. But most of the time I’m kidding myself when I give up on things. If it’s the courageous decision to move on, move on. If it’s the courageous decision to keep going, keep going.
Never give up. You can make it. It is possible. Don’t listen to me: listen to Churchill:
“Never give in, never give in, never, never, never, never—in nothing, great or small, large or petty—never give in except to convictions of honor and good sense.”
– Winston Churchill
Now close this tab and go and achieve something amazing.
Share
More articles
How to lead your life
Some of us might say that we aren’t leaders. However, we are all leaders of at least one thing: our own lives. We determine the way that we should go, what we spend today doing. If we allow our life to happen to us, then the our lives will be determined by the whim of others and not ourselves.
It’s quite possible for us to lead our lives without actually leading our lives, so in 2017 I’ve decided to try to do better at living a life that counts.
As a first step, I spent the first part of the week trying to simplify my life down to the core essence of what I want to focus on. Here’s my list today:
- Build relationship with Ellie and the kids.
- Continue my current fitness training regime.
- Try to live according to the teaching of Jesus in my community. This is separate from what people might think of as “being a Christian”, but that’s a topic for another time.
- Work to create systems that make people’s lives better. I’m currently doing this for money for various organisations, and by creating fun video games.
- Teach and train people how to lead others and be amazing - that’s why I’m writing this post.
- Improve my writing skills and range.
If something I’m doing doesn’t fit into this list, then I’ve decided to work towards removing it from my life.
More than ever in this present age of mass distraction, our headspace is the key limiting factor to productivity and achievement. It’s a resource to be managed and conserved. I’m only a week in, but have benefited hugely from the reduced focus already, and my job satisfaction is much improved.
If you made a list such as the one above, what would be on it? What should we remove in order to conserve headspace, and to focus on what counts?
Read moreHow to add live code reload to your game
Adding live code reloading is one of the best things I did when working on my current game, Sol Trader.
Live code reload reduces our debug loop down to milliseconds. Now when I recompile the game, it notices I’ve done so and loads new code whilst the game is still running. Here’s a demo:
Thanks to Casey Muratori (again) and his excellent Handmade Hero series for teaching me the basics, and to this github project for demonstrating how to do it in OSX, my development platform of choice.
There are a few architecture changes that we’ll need in order to put this feature in our code. Here’s how I did it.
Splitting our game code from our platform
Sol Trader now has a clearly defined platform layer, separate from the game. The game code itself knows nothing about the platform at all and it passed in everything it needs in order to calculate what’s next. Here’s the method that we call to run a game loop:
bool gameUpdateAndRender(Memory* memory, v2i screenDim, Input* input);
The update and render call takes some Memory
, the screen dimensions and the input that we received this frame from the player. It is the job of this method to render the next frame to the screen, in under 16ms.
Memory
is just a big chunk of raw memory allocated by the platform layer at the start of the game. The gameUpdateAndRender
function is free to do what it likes with this space. It’s important to note that it’s persistent across live reloads which means that all state should be saved here. The game is not allowed to allocate any memory itself, it has to use the memory given to it.
gameUpdateAndRender
actually is implemented as a call into a shared library (a DLL on windows, or a dylib on Linux/OSX) using a #define trick I learnt from Handmade Hero:
// Platform.h
#define GAME_UPDATE_AND_RENDER(name) bool name(Memory* memory, v2i screenDim, Input* input)
typedef GAME_UPDATE_AND_RENDER(GameUpdateAndRender);
// Game.cpp
extern "C" GAME_UPDATE_AND_RENDER(GAME_updateAndRender) {
// game code here
}
(We need the extern "C"
here to stop the compiler from mangling the name, so we can find it in the shared library.)
Running the game code
This is a cut down OSX version of the platform layer I’m using. Similar code exists for other platforms:
// Platform.cpp
void OSX_loadGameCode(GameCode* game, char const* path) {
if ((game->code = dlopen(path, RTLD_LAZY | RTLD_GLOBAL))) {
game->updateAndRender = (sol::GameUpdateAndRender*)dlsym(game->code, "gameUpdateAndRender");
game->lastWriteTime = OSX_lastWriteTime(gameCodePath);
}
}
void main() {
Memory memory;
allocateMemory(&memory);
initOpenGL();
v2i screenDim = createGameWindow();
GameCode game;
char const* gameCodePath = "soltrader.dylib";
OSX_loadGameCode(game, gameCodePath);
// Main loop
while (!quit) {
Input newInput;
getInput(&newInput);
// Check if the game has been recompiled
time_t newWriteTime = OSX_lastWriteTime(gameCodePath);
if (newWriteTime != game->lastWriteTime) {
OSX_unloadGameCode(&game);
OSX_loadGameCode(&game, gameCodePath);
}
if (game.updateAndRender) {
game.updateAndRender(&memory, screenDim, &newInput);
}
}
}
At the heart of it, it’s a standard game loop. We first allocate enough memory using one big alloc
call at the beginning. This is all platform specific code, so it’s ok to use OSX, Linux or Windows specific calls here. We figure out our screen dimensions from the platform, create a window, and initialise OpenGL or whatever graphics library we’re using.
Then we load the code using dlopen
and if that succeeds, we find the gameUpdateAndRender
function and save the location. In the main loop, assuming that all worked, we call the saved function with the info it needs to render the frame.
Building the shared library
Here’s how the build.sh
script looks:
// Build the shared library
$CC $CFLAGS -dynamiclib ../src/Unity.cpp -o ../libsol.dylib $LIBS
// Build the executable
$CC $CFLAGS -o ../$EXE ../src/platforms/sdl2/Application.cpp $LIBS
We build the shared library containing only the game code, not the platform code. We then use the platform code to load and run the shared game library.
Summary: everyone should have live code reload
This is an amazing way to develop games. For too long in the past I have sat watching a long compile, then ploughed through the game from the main menu, to find the bug I’m trying to fix, only to find that I’ve made a stupid error and have to start again. We need to find fun as fast as possible - anything we can do to reduce the debug loop is a good thing.
Live code reload also does away with much of the need to use a scripting language (fast feedback). I don’t have any designers who don’t write C (it’s just me!) so I haven’t implemented one for this game. I also don’t need any GUI configuration files for layout, it’s all just implemented in C with live code reload for positioning tweaks.
Trust me: once you’ve tried it, you’ll never go back.
Read moreThe difference review and planning makes to indie development
I’m currently in a pretty good groove working on Sol Trader’s development. Due to other work commitments I can only manage 2-3 days a week on it at the moment, but my productivity is pretty much at an all time high.
One of the things that’s helped me is reading a great article on how to motivate ourselves as indie game developers. One of the most helpful tips was to keep a list of all our accomplishments week by week, and to plan the week ahead. It sounds simple, but it’s already made a big difference to my work. Here’s how I do it.
List accomplishments from last week
On Monday morning I record a list of what I’ve done using Notes on my Mac. I trawl through todo list items, Github commits and my marketing plan. Here’s Sol Trader’s from last week:
## Time last week 1 full day plus 4 evenings ## Last week - code: * Switched the renderer coordinate system around * Added planet rendering * Refactored rendering out of platform into game code * Added normal maps and sun angle * Show the correct planet on the background * Fill essential vacancies * Started work on entity sheet pane - loads of refactoring on it * Refactored all relationships to separate component * Custom rendering of ship images on GUI * Auto-reload shaders on Cmd-R * Tweaked the bloom code loads * Introduced higher resolution planets * Moved premultiply of alpha out to script on game start * Fixed last seen location for friends * Started ship hiring components ## Last week - marketing: * Live stream * Montage: focus * Weekly promotional thread on /r/indiegaming * Blog post on bloom * Facebook ad on new main menu screenshot
This was enormously motivating for me: despite only giving half a week to the game I got a huge amount of development done! It’s worth really celebrating this achievement.
Plan the upcoming week
I then work out how much time I have to work on the game next week. Then I make a realistic list on the goals I want to achieve in the week, with loose estimates, based on the amount of time I have. Here my list for this week - as I have about 3.5 days, I’ve given myself 2.5 days of goals for the week, plus a stretch goal.
## Time this week 3 days plus 1 evening ## Next week goals [X] finish relational currency work: 0.5d [ ] Banks and borrowing money: first pass - 1d [ ] Ability to hire ships: first pass - 0.5d [X] Marketing: livestream - 0.25d [X] Marketing: Montage: quote on motivation [X] Marketing: Weekly promotional thread on /r/indiegaming [ ] Marketing: Blog post: how I plan my week - 0.25d [ ] Marketing: Friday Newsletter with a roundup of latest work - 0.25d ## Stretch goal: Launching a ship - basics of space back in - 1d
It’s very important to pace ourselves here. We should not plan more work that we can realistically do. If we easily achieved last week’s goals, then we should plan a little more: if we didn’t achieve them, then we should ask ourselves why and do a thorough review. Also, we need to make sure we get enough sleep. I definitely need 7-8 hours a day - otherwise when I work on my game I end up subtracting value rather than adding it, and short-changing my family and other commitments.
This week, I’m already ahead of myself. I’ve almost finished adding in banks and borrowing money (the final thing I need to add is handling a loan default, which I’ll do this morning) and I should get ship hiring in today as well. The quote on motivation was popular on Facebook, which is encouraging, and the live stream went well. That leaves most of a day to get the newsletter and the ship launching code back in, which is my stretch goal.
Summary
Sometimes indie development can feel rather like moving the game from 46.001% to 46.002% completed each day. Without a clear measurement of progress, it can be a real struggle. I’ve found this process of review and planning enormously helpful. What methods do you use in order to stay motivated?
Read more"Project velocity is a useless metric." Discuss.
The conversation
Chris: “Velocity is useless, and at worst leads product people to focus on a number rather than their features. The only thing that is important is ‘will this (small) story X be done by Y.’ and the PM saying: ‘how confident are you in that?’ and ‘what can I do to help you become more confident?’”
Matt: “So I do disagree with that somewhat. I wouldn’t call velocity useless, but it’s a signal something is wrong.”
Chris: “Ah, ok. Interesting.”
Matt: “You can use velocity to build trust, where stakeholders are used to estimates on the scale of months, and slippages on the scale of weeks. Organisations in that kind of a state can benefit from a bit more transparency. It builds trust BUT the need for it shows you that you don’t have the trust.”
Chris: “I get that it can be used as training wheels. I prefer to count number of shipped features, but that’s an equivalent metric. I don’t like focusing on a number: I’d prefer to demo running software, rather than aggregate features artificially.”
Matt: “Ultimately, almost all the questions like ‘when is it going to be done?’ are asked because of a lack of trust.”
Chris: “Not all, in my opinion. It’s reasonable to ask when something will be done by. Roughly. If you’re asking for the right reasons.”
Matt: “Yes, sometimes you have fixed dates. What are the right reasons?”
Chris: “External reasons, not internal ones. Like ‘the Olympics.’”
Matt: “Right, but why do we need to know whether this little story X will be done by this week, when the Olympics are 6 months away still?”
Chris: “Because sometimes there are external things that depend on little stories. When something is live, the deadlines get smaller and more frequent. External deadlines are sometimes imposed, even for small stories.”
Matt Wynne: “Specifically, I’m saying stakeholders tend not to trust: 1) you’re working on the highest priority things 2) you’re working as hard as you could be. My experience is that when you really get that trust, stakeholders stop asking for estimates.”
Matt: “Even with a fixed deadline, the project owners share the responsibility for finding the scope that’s possible.”
Chris: “My experience on my current project is we hardly ever get asked when something will be done.”
Matt: “Perfect.”
Chris: “…and velocity is mostly ignored. The general sense of “less stories this week” isn’t ignored though, and sensible questions are asked.”
Matt: “Aha, so that’s another use for velocity: a signal that the team have built up technical debt that’s starting to drag them down.”
Chris: “Perhaps so. In this case its more likely to be external blockers.”
Matt: “Yeah, and there are other better signals for that.”
Chris: “I regard the infatuation with the ‘numbers’ as uselessly reductionistic.”
Matt: “Right. This is where it starts to bite. People lose sight of the point of the numbers.”
Matt: “(in mock jubilation) ‘our velocity was 28.7 this week, up 0.3 from last week!’”
Chris: “(in response) ‘W00t! Let’s spend 1.73 hours each next week sorting out technical debt. My spreadsheet tells us we’re allowed to!’”
Chris: “If you’re going to track anything, track cycle time. But do it informally even so.”
Matt: “Have I convinced you yet that velocity isn’t useless?”
Chris: “No. Well… velocity is a loaded term.”
Matt: “Points / week.”
Chris: “Useless. Shipped features a week == useful training wheels.”
Matt: “Ah ok.”
Chris: “I can’t be bothered to explain points to stakeholders; they don’t care.”
Matt: “So that’s my thing: 1 story = 1 point. You can’t have it any other way: then all your stories have to be small if you want a fast velocity! It’s a game…”
Chris: “Fine. I like that game. Substitute ‘story’ for ‘point’ everwhere and I’m happy. List the features you delivered to stakeholders, not the points.”
Matt: “So take a team who are already doing all of the scrum dances. What are you going to change first?”
Chris: “Yeah, I can see using that as a transition exercise. I’m being idealistic, of course. It’s not how you’d transition a team. Change things slowly and get buy in everywhere for apparently superfluous changes.”
Matt: “I have watched 8 people spend a whole morning fretting over estimating stories, then they spent another half day estimating all the tasks in hours.”
Chris: “Oh yeah, I used to do that.”
Matt: “Me too. I wrote this post basically for that team.”
Matt: “Oh, and here’s something else I wrote on BDD and velocity, too.”
Chris: “(After reading the posts) Good articles. I like the idea of countings scenarios not points. I don’t consider CFDs (Cumulative Flow Diagrams) to have no value. The value is same as Kanban - it lies in in identifying blockages.”
Matt: “The nice thing (about CFDs) is you can see how your flow is changing over time. A kanban board is just a snapshot.”
Chris: “True. It’s the same learning though. A CFD will show your learning history, which is useful for seperate reasons.”
The verdict
True to all good conversations, this one ended with me having a slightly different point of view to the one I came in with:
Velocity isn’t entirely useless. Matt’s game of “1 story = 1 point” will help a team to make a move away from an overreliance on a Big Agile approach, where a meaningless metric might hold sway over a common sense look at what’s really going on inside a team.
Showing stakeholders a velocity metric each week to two helps to build trust in an organisation that’s used to disappointment. However, an excessive thirst for these numbers from the business shows that a team has some way to go to earn that trust.
Finally, there’s nothing quite like shipping working software to gain people’s confidence. I prefer to provide a bulleted list of the new things that have been added, as opposed to wasting time over-analysing and reducing the week’s changes into one potentially misleading number.
What’s your opinion? Do you favour the velocity metric, or is it something you can do without?
Read moreDelegated tasks are a team anti-pattern
“Jane, I’d like you to phone up the recruiter, and tell them we need a new agency person. Don’t use Jim from Acme Recruitment again, you didn’t get very far with him last time. Make sure you book whoever it is in for a week to work with us as a trial, like last time. That worked well.”
“Jane, can you find us a great developer for the new website we mentioned in standup last week? Let me know if you need help.”
Which is better?
Goals, not tasks
How about we give our team goals, not tasks? Let them shoot for something, and work out their own tasks, rather than giving them a simple list of things to do. Goals allow people to apply their own creativity and their own flair to a solution, and the end result will be stamped with their individuality.
When learning a new skill, people need direction and tasks to follow. Matt Wynne recently re-iterated the classic Su-Ha-Ri model of learning, where we start with very clear forms to follow, then break those forms as we try new things, then advanced to a place where we no longer need the forms at all. At first, we need to work closely with people, and show them the tasks we perform to get something done. Note that this is quite different to giving people a long list of tasks to complete to ‘learn something.’
Whenever we give something away, there’s a risk that it won’t be done in quite the way that we would like. The simple fact is: no, it won’t. But assuming we’ve not overstretched someone, there’s a good chance they’ll get the job done at least 80% as well as we could have. And good people will cope with being stretched much further than we think.
There’s delegation, then there’s abdication
When we take goal setting too far, we just tend to stop giving people goals altogether and let them figure out their own jobs. This is dangerous: the best people don’t need managing, but they do need leading. Our role as a leader is to paint an exciting vision of the future, and then let our team figure out how to get there.
Micromanagement has many levels
It’s quite possible to micro-manage without realising it. We might think we’re not micro-managing because we’re not telling people exactly how to do something. However if we’re leaving little room for doubt in our own minds, and creativity in theirs, then our team will feel less able to apply their own skills and talents to the problem. They’ll get up feeling discouraged and insignificant.
Ultimately it comes down to trust, and fear. How much do we trust our people to get the job done? How much do we fear losing control?
The first step to fighting a task-oriented tendency is to realise it’s probably not a problem with our team members, but with us.
Read more